Differentiate naming function, var, helper, etc
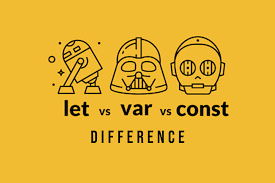
-
Functions/Methods:
- Use camelCase for function names.
-
Use descriptive names that indicate the purpose of the function.
javascriptCopy code function calculateTotalPrice() { // function logic here }
-
Variables:
- Use camelCase for variable names.
-
Choose descriptive names that convey the meaning of the variable.
javascriptCopy code let studentName = "John";
-
Constants:
- Use uppercase letters and underscores for constants.
-
Constants are usually declared at the top of the file or in a separate file.
javascriptCopy code const MAX_VALUE = 100;
-
Components (in React or Vue):
- Use PascalCase for component names.
-
Component names should be nouns and represent a specific UI element.
javascriptCopy code // React class MyComponent extends React.Component { // component logic here } // Vue export default { name: 'MyComponent', // component logic here }
-
Helpers/Utilities:
- Use camelCase for utility function names.
-
Include descriptive names indicating the utility's purpose.
javascriptCopy code function formatDate(date) { // utility logic here }
-
Classes:
- Use PascalCase for class names.
-
Class names should be nouns and represent a blueprint for objects.
javascriptCopy code class Car { // class logic here }
-
Booleans:
-
Use names that make it clear the variable is a boolean.
javascriptCopy code let isEnabled = true;
-
-
Callbacks:
-
Use descriptive names for callback functions.
javascriptCopy code fetchData((data) => { // callback logic here });
-
Remember that consistency within a codebase is crucial. Choose a set of conventions that make sense for your team or project and stick to them. This will make your codebase more readable and maintainable for you and your collaborators.